클로드로 한글 문서 읽기
1. 한글 문서 읽기
한국에서는 한글 문서가 많이 사용됩니다. 한글 문서를 읽고 쓸 수 있다면 이전에 진행했던 보고서, 이전에 진행했던 행사 등에 대한 보고서를 참고하여 새로운 보고서로 정리를 한다던지, 앞서 배운 엑셀로 정리를 한다던지 하는 것이 손쉽게 가능할 것입니다. 여기서는 olefile
이라는 라이브러리를 사용하여 한글문서를 읽는 방법에 대해 알아보도록 하겠습니다. pip install olefile
을 통해 설치합니다.
2. 한글 문서 작성
아래와 같이 한글 문서 내에 작성해서 test폴더 아래 test.hwp파일로 생성합니다.
hello world!
한글 문서 읽기!
hello world!
한글 문서 읽기!
3. 파일 생성
아래와 같이 작성합니다. 이번 챕터에서는 Python 코드만 제공합니다. 파일 이름, config 파일 등은 앞에 챕터를 통해 확인해주세요.
from mcp.server.fastmcp import FastMCP
# MCP 서버 생성
mcp = FastMCP(name="read_hangle", host="127.0.0.1", port=5011, timeout=30)
# 한글 문서 읽기
@mcp.tool()
def read_hwp(file_name: str) -> str:
"""한글 문서(.hwp)를 읽어 텍스트로 반환합니다.
olefile 라이브러리를 사용하여 한글 문서의 텍스트 내용을 추출합니다.
Args:
file_name (str): 읽을 한글 문서의 이름
예: 'document.hwp'
Returns:
str: 한글 문서에서 추출한 텍스트 내용 또는 오류 메시지
"""
import os
import olefile
# pip install olefile 하셔야 합니다.
# 상대 경로인 경우 현재 디렉토리 기준으로 절대 경로 변환
file_path = os.path.join("c:/test", file_name)
try:
# 한글 파일 열기
if not olefile.isOleFile(file_path):
return f"오류: '{file_path}'는 올바른 한글 문서 형식이 아닙니다."
ole = olefile.OleFileIO(file_path)
# 텍스트 스트림 읽기 시도
if ole.exists("PrvText"):
text_stream = ole.openstream("PrvText")
text_data = text_stream.read().decode("utf-16-le", errors="replace")
ole.close()
return text_data
# 미리보기 텍스트가 없는 경우 대안 시도
elif ole.exists("BodyText/Section0"):
section = ole.openstream("BodyText/Section0")
data = section.read()
ole.close()
# 간단한 텍스트 추출 (완전하지 않을 수 있음)
result = ""
for i in range(0, len(data), 2):
if i + 1 < len(data):
code = data[i] + (data[i + 1] << 8)
if code > 31 and code < 127:
result += chr(code)
elif code > 255:
result += chr(code)
else:
result += " "
return result
else:
ole.close()
return "텍스트 내용을 추출할 수 없습니다. 지원되지 않는 한글 문서 형식일 수 있습니다."
except Exception as e:
return f"한글 문서 읽기 오류: {str(e)}"
# 서버 실행
mcp.run()
from mcp.server.fastmcp import FastMCP
# MCP 서버 생성
mcp = FastMCP(name="read_hangle", host="127.0.0.1", port=5011, timeout=30)
# 한글 문서 읽기
@mcp.tool()
def read_hwp(file_name: str) -> str:
"""한글 문서(.hwp)를 읽어 텍스트로 반환합니다.
olefile 라이브러리를 사용하여 한글 문서의 텍스트 내용을 추출합니다.
Args:
file_name (str): 읽을 한글 문서의 이름
예: 'document.hwp'
Returns:
str: 한글 문서에서 추출한 텍스트 내용 또는 오류 메시지
"""
import os
import olefile
# pip install olefile 하셔야 합니다.
# 상대 경로인 경우 현재 디렉토리 기준으로 절대 경로 변환
file_path = os.path.join("c:/test", file_name)
try:
# 한글 파일 열기
if not olefile.isOleFile(file_path):
return f"오류: '{file_path}'는 올바른 한글 문서 형식이 아닙니다."
ole = olefile.OleFileIO(file_path)
# 텍스트 스트림 읽기 시도
if ole.exists("PrvText"):
text_stream = ole.openstream("PrvText")
text_data = text_stream.read().decode("utf-16-le", errors="replace")
ole.close()
return text_data
# 미리보기 텍스트가 없는 경우 대안 시도
elif ole.exists("BodyText/Section0"):
section = ole.openstream("BodyText/Section0")
data = section.read()
ole.close()
# 간단한 텍스트 추출 (완전하지 않을 수 있음)
result = ""
for i in range(0, len(data), 2):
if i + 1 < len(data):
code = data[i] + (data[i + 1] << 8)
if code > 31 and code < 127:
result += chr(code)
elif code > 255:
result += chr(code)
else:
result += " "
return result
else:
ole.close()
return "텍스트 내용을 추출할 수 없습니다. 지원되지 않는 한글 문서 형식일 수 있습니다."
except Exception as e:
return f"한글 문서 읽기 오류: {str(e)}"
# 서버 실행
mcp.run()
4. 실행화면
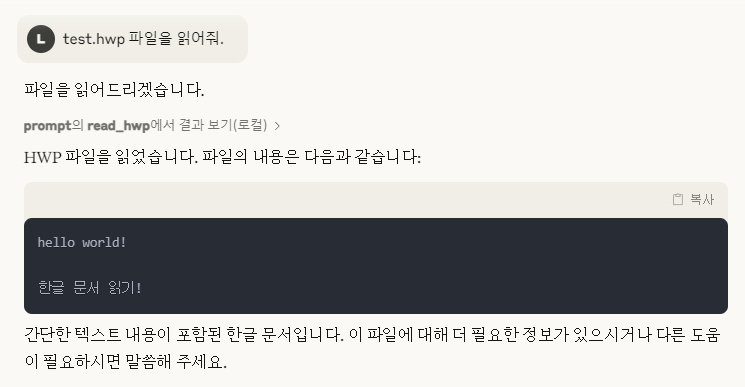