실습환경 세팅
1. 실습환경 세팅
실습 환경은 jupyter notebook을 사용합니다. 아래 링크를 클릭하여 실습을 진행해 주세요. 꼭 jupyter notebook이 아니라 여러분이 친숙하게 사용하시는 환경을 사용하셔도 무방합니다. 여기서 구축된 jupyter notebook을 사용하는 이유는 아래와 같습니다.
- 로그인 없이 사용할 수 있어야 합니다.
- 실습 환경을 구축하는데 시간이 소요되지 않아야 합니다.
- 노트북을 저장해서 언제든지 열어볼 수 있어야 합니다. VSCode와 같은 곳에서도 열어볼 수 있어요.
- 다시 실행할 수 있어야 합니다.
- 언제든지 다시 접속할 수 있어야 합니다.
2. 실습 환경 사용법
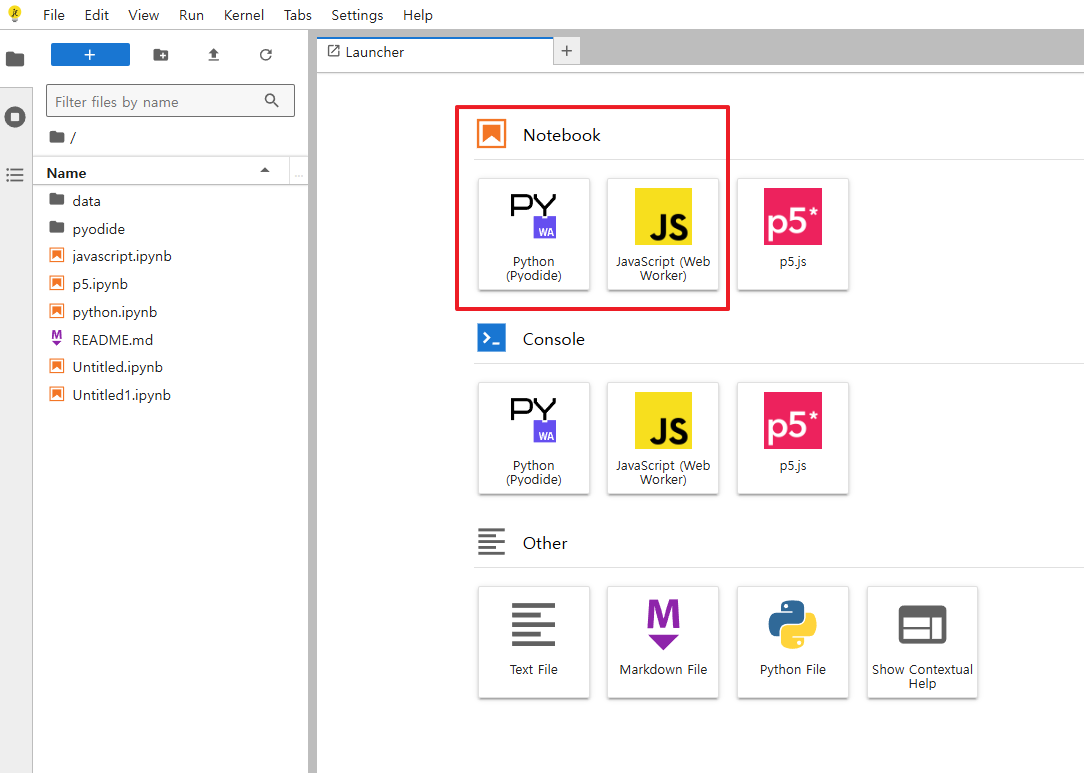
노트북에 접속하셔서 원하시는 언어를 클릭해주세요. 그러면 해당 언어의 실습 환경이 열립니다.
- 셀을 클릭하면 코드를 수정할 수 있습니다. 이전 코드도 수정이 가능합니다.
- 셀을 실행하려면
Shift + Enter
를 누르면 됩니다. 만약 아래 셀이 더 생성되지 않게 하려면Ctrl + Enter
를 누르면 됩니다. - 셀 옆에 쓰레기통 버튼을 누르면 해당 셀이 삭제됩니다.
- 셀의 위치는 마우스로 드래그해서 이동할 수 있습니다. 오른쪽에 위, 아래 버튼을 눌러 이동할 수도 있습니다.
3. 실습 환경 종료 및 다운로드
File 탭을 눌러 해당 노트북을 다운로드 받을 수 있습니다. 해당 파일은 브라우저에 저장되는 방식이기 때문에 브라우저를 종료하셔도 같은 컴퓨터라고 한다면 파일이 남아있습니다.